20 Node.js modules you need to know
We present our pick of the Node.js modules that will help you during the development of web applications and more.
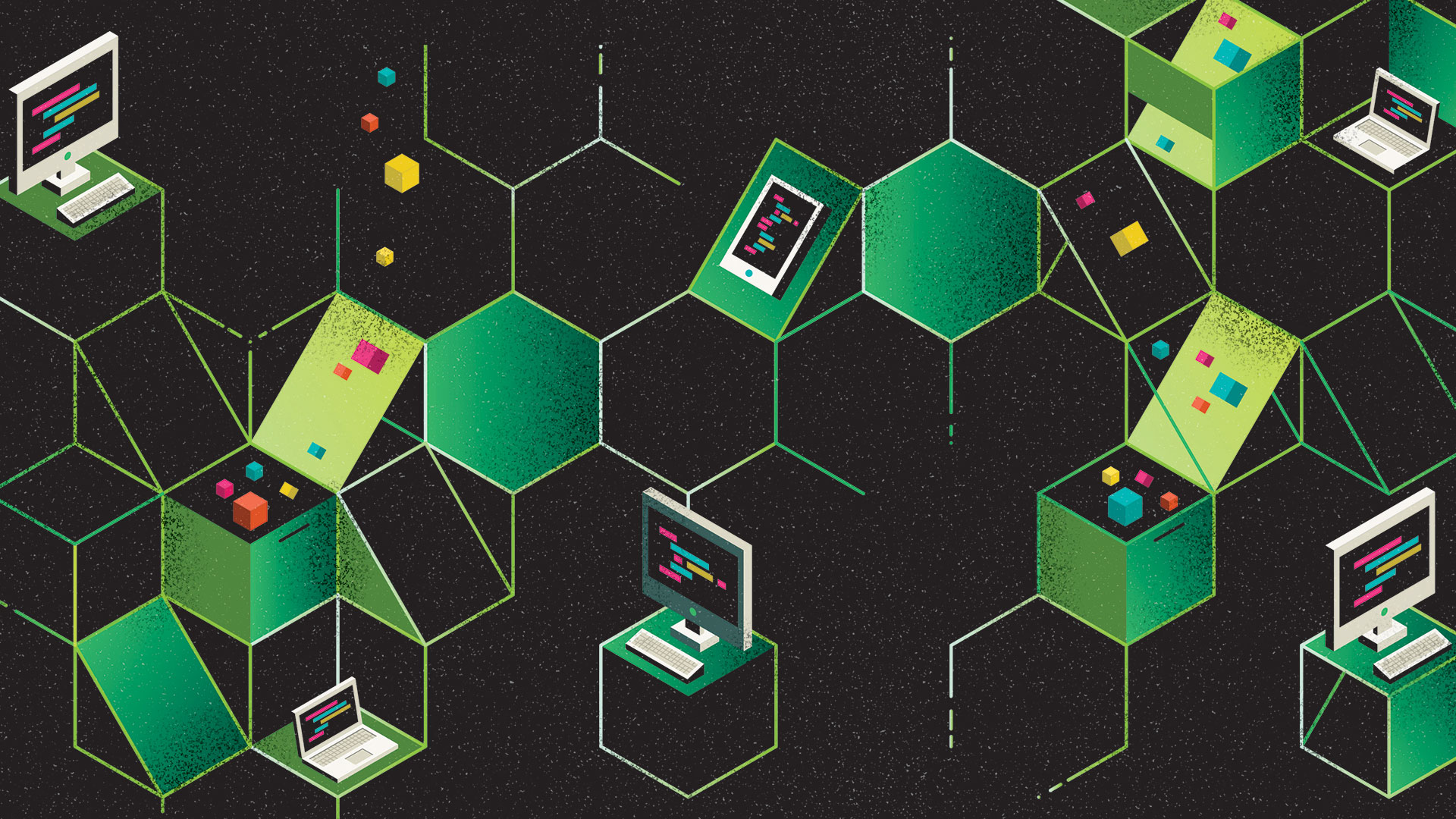
Over recent years Node.js has become more and more popular. It is now often used for developing the server side of web applications, or in general during the development process. At the time of writing, the homepage of npm – the package manager for Node.js – lists over a quarter of a million modules.
I've put together a list of the ones I find useful in my daily work as a web and software developer, from image manipulation, string validation and PDF generation to minification, logging and the creation of command line applications.
Working with images
01. Manipulate images
GraphicsMagick and ImageMagick are two popular tools for creating, editing, composing and converting images. Thanks to the Node.js module gm you can use both tools directly from within your JavaScript code. The module supports all the typical image operations – resizing, clipping and encoding to name just a few.
const gm = require('gm');
gm('/path/to/image.jpg')
.resize(500, 250)
.autoOrient()
.write(response, error => {});
02. Process images
Sharp is based on the ultra-fast libvips image processing library, and claims to be four to five times faster than ImageMagick or GraphicsMagick when it comes to compressing and resizing images. It supports JPEG, PNG, WebP, TIFF, GIF and SVG images, and outputs data into either JPEG, PNG, WebP or uncompressed raw pixel streams.
03. Generate sprite sheets
Sprite sheets are bitmap files that contain many different small images (for example icons), and they are often used to reduce the overhead of downloading images and speed up overall page load. Generating sprite sheets manually is very cumbersome, but with spritesmith you can automate the process. This module takes a folder as input and combines all the images in it into one sprite sheet. It also generates a JSON file that contains all the coordinates for each of the images in the resulting image, which you can directly copy in your CSS code.
Dates, strings, colours
04. Format dates
The standard JavaScript API already comes with the Date object for working with dates and times. However, this object is not very user-friendly when it comes to printing and formatting dates. On the other hand, Moment.js offers a clean and fluid API, and the resulting code is very readable and easy to understand.
moment()
.add(7, 'days')
.subtract(1, 'months')
.year(2009)
.hours(0)
.minutes(0)
.seconds(0);
In addition, there is an add-on available for parsing and formatting dates in different time zones.
Get top Black Friday deals sent straight to your inbox: Sign up now!
We curate the best offers on creative kit and give our expert recommendations to save you time this Black Friday. Upgrade your setup for less with Creative Bloq.
05. Validate strings
When providing forms on a web page, you always should validate the values the user inputs – not only on the client-side, but also on the server-side to prevent malicious data. A module that can help you here is validator.js. It provides several methods for validating strings, from isEmail() and isURL() to isMobilePhone() or isCreditCard(), plus you can use it on the server- and the client-side.
06. Work with colour values
Converting colour values from one format into another is one of the tasks every frontend developer needs to do once in a while. TinyColor2 takes care of this programmatically, and it's available for Node.js as well as for browsers. It provides a set of conversion methods (e.g. toHexString(), toRGBString()), as well as methods for all sorts of colour operations (e.g. lighten(), saturate(), complement()).
Working with different formats
07. Generate PDF files
You want to dynamically generate PDF files? Then PDFKit is the module you are looking for. It supports embedding font types, embedding images and the definition of vector graphics, either programmatically (using a Canvas-like API) or by specifying SVG paths. Furthermore, you can define links, include notes, highlight text and more. The best way to start is the interactive browser demo, which is available here.
08. Process HTML files
Ever wanted to process HTML code on the server side and missed the jQuery utility methods? ThenCheerio is the answer. Although it implements only a subset of the core jQuery library, it makes processing HTML on the server side much easier. It is built on top of the htmlparser2 module, an HTML, XML and RSS parser. Plus, according to benchmarks, it's eight times faster than jsdom, another module for working with the DOM on the server side.
09. Process CSV files
The CSV (comma-separated values) format is often used when interchanging table-based data. For example, Microsoft Excel allows you to export or import your data in that format. node-cvg simplifies the process of working with CSV data in JavaScript, and provides functionalities for generating, parsing, transforming and stringifying CSV. It comes with a callback API, a stream API and a synchronous API, so you can choose the style you prefer.
10. Process markdown files
Markdown is a popular format when creating content for the web. If you ever wanted to process markdown content programmatically (i.e. write your own markdown editor), marked is worth a look. It takes a string of markdown code as input and outputs the appropriate HTML code. It is even possible to further customise that HTML output by providing custom renderers.
Next page: Explore the best minifiers and utility modules
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1