Speed up performance with Vue.JS
Learn how to manage state and get faster load times with lazy loading.
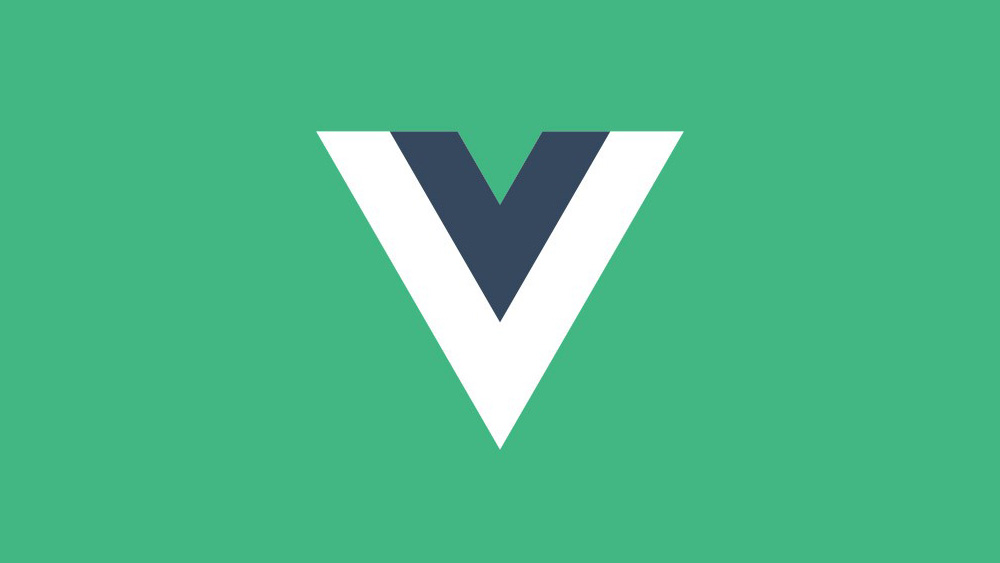
As with any component-based library, managing state in Vue can be tricky. While the application is small, it’s possible to keep things in sync by emitting events when values change. However, this can get brittle and prone to errors as the application grows, so it may be better to get a more centralised solution in from the start.
If you’re familiar with Flux and Redux, Vuex works much the same. State is held in one centralised location and is linked to the main Vue application. Everything that happens within the application is reflected somewhere within that state. Components can select what information is relevant to them and be notified if it changes, much like if it was part of its internal state.
Changing state
A Vuex store is made up of four things – the state, getters, mutations and actions. The state is a single object that holds all the necessary data for the entire application. The way this object gets structured depends on the project, but would typically hold at least one value for each view.
Getters work like computed properties do inside components. Their value is derived from the state and any parameters passed into it. They can be used to filter lists without having to duplicate that logic inside every component that uses that list.
The state cannot be edited directly. Any updates must be performed through mutation methods supplied inside the store. These are usually simple actions that perform one change at a time. Each mutation method receives the state as an argument, which is then updated with the values needed to change.
Performing mutations
Mutations need to be synchronous in order for Vuex to understand what has changed. For asynchronous logic – like a server call – actions can be used instead. Actions can return Promises, which lets Vuex know that the result will change in the future as well as enabling developers to chain actions together.
To perform a mutation, they have to be committed to the store by calling commit() and passing the name of the mutation method required. Actions need to be dispatched in a similar way with dispatch().
Daily design news, reviews, how-tos and more, as picked by the editors.
It’s good practice to have actions commit mutations rather than committing them manually. That way, all updating logic is held together in the same place. Components can then dispatch the actions directly, so long as they are mapped using the mapActions() method supplied by Vuex.
To avoid overcomplicating things, the store can also be broken up into individual modules that look after their own slice of the state.
Each module can register its own state, getters, mutations and actions. State is combined between each module and grouped by their module name, in much the same way as combineReducers() works within Redux.pport.
Speeding up the first load
By default, the entire contents of the application end up inside one JavaScript file, which can result in a slow page load. A lot of that content is never used on the first screen the user visits. Instead it can be split off from the main bundle and loaded in as and when needed. Vue makes this process incredibly simple to set up, as vue-router has built-in support for lazy loading.
const AsyncAbout = () => import(‘./About.vue’);
const router = new VueRouter({
routes: [
{ path: ‘/about, component: AsyncAbout }
] })
Vue supports using dynamic imports to define components. These return Promises, which resolve to the component itself. The router can then use that component to render the page like normal. These work alongside code splitting built in to webpack, which makes it possible to use features like magic comments to define how components should be split.
Related articles:
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
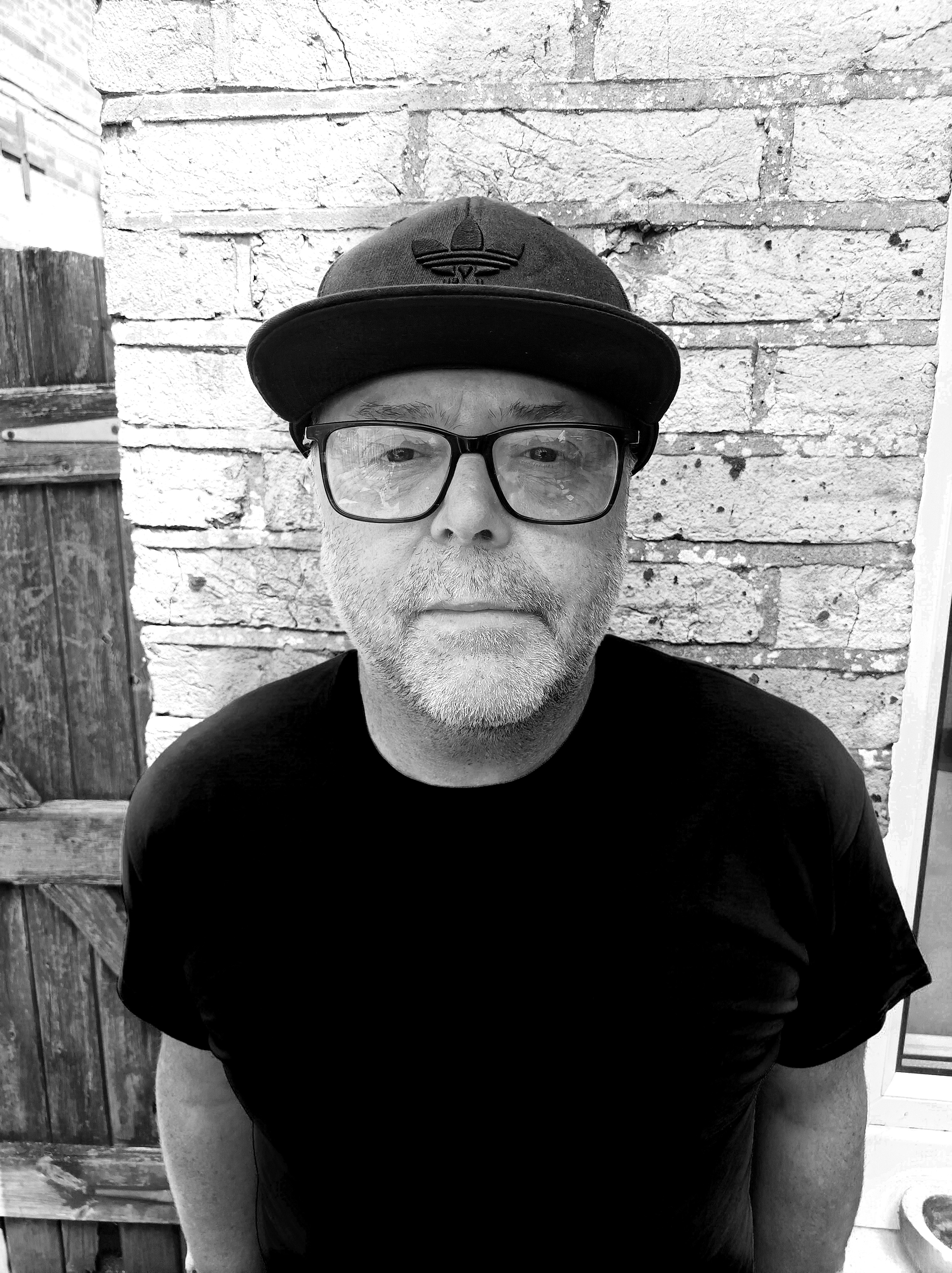
Steven Jenkins is a freelance content creator who has worked in the creative industries for over 20 years. The web and design are in his blood. He started out as a web designer before becoming the editor of Web Designer magazine and later net magazine. Loud guitars, AFC Bournemouth, Photoshop, CSS, and trying to save the world take up the rest of this time.