Get started with the Genesis Framework
Carrie Dils introduces the Genesis Framework and explains how to get started working with child themes.
In the WordPress world, frameworks tend to refer to some sort of starter or boilerplate code. By starting new theme build projects with a framework, you save development time, reduce repetitive tasks, and generally give yourself a head-start on projects. While all of that is true for Genesis, in this case the word 'framework' can be a little misleading.
The work of StudioPress, the Genesis Framework is simply a parent theme, albeit a pretty pimped out one. It's a toolbox for building child themes, which gives you a consistent output of HTML markup and a basic CSS style sheet to use as a starting point for your customisations. It's easy to assume such a robust theme will be bloated or full of unnecessary code, but Genesis is surprisingly lightweight (the entire zip is just over 400KB). It's coded (and documented) very well, extremely secure, and integrates schema.org with its HTML5 markup, giving users an SEO advantage right out of the gate.
Genesis includes pre-built components for all of your standard web layouts – navigation menus, headers, sidebars, footers and so on. It's like a set of Lego – its modular structure enables you to add, move or remove anything.
Building blocks
Here's where Genesis differs from other parent themes, instead of relying heavily on templates, child theming with Genesis is primarily about working with action hooks and filters. Genesis provides a base from which all of your Lego blocks can be shifted, replaced or modified, without ever touching a template file (although you certainly can use templates). The most basic child theme only requires a style sheet and a 'functions.php' file.
In short, Genesis is incredibly flexible and poses no limitations on development. If it can be done with WordPress, it can be done in Genesis.
File structure
At some point, even if you don't call yourself a developer, you'll want to customise your theme in some way. When the moment comes for you to drop in a code snippet or write your own function, your work will go more smoothly if you have a conceptual overview of the framework.
If you unzip Genesis and look at the file structure, you'll see the root files are really no different from what you'd expect to find in any WordPress theme: there are a dozen or so standard template files and a style sheet. From there, various folders contain files for specific bits of functionality. The magic file is in the '/lib/' folder: 'framework.php':
function genesis() {
get_header();
do_action( 'genesis_before_content_sidebar_wrap' );
genesis_markup( array(
'html5' => '<div %s>',
'xhtml' => '<div id="content-sidebar-wrap">',
'context' => 'content-sidebar-wrap',
) );
do_action( 'genesis_before_content' );
genesis_markup( array(
'html5' => '<main %s>',
'xhtml' => '<div id="content" class="hfeed">',
'context' => 'content',
) );
do_action( 'genesis_before_loop' );
do_action( 'genesis_loop' );
do_action( 'genesis_after_loop' );
genesis_markup( array(
'html5' => '</main>', //* end .content
'xhtml' => '</div>', //* end #content
) );
do_action( 'genesis_after_content' );
echo '</div>'; //* end .content-sidebar-wrap or #content-sidebar-wrap
do_action( 'genesis_after_content_sidebar_wrap' );
get_footer();
}
This file is the heart of the Genesis Framework and is really the only part that can't be changed. It's the foundation upon which everything else is built. The genesis() function declared here is called from nearly every standard template file. When this function is called, 'framework.php' is loaded and all the code within this function is processed.
If you've done any theming or customising in WordPress, the get_header() and get_footer() functions probably look familiar. Those call in the 'header.php' and 'footer.php' files respectively. In-between those functions is the basic structure of every page, including the Loop (which displays the current post or page), some basic HTML markup, and seven action hooks. If you're curious and want to follow the rabbit hole, do a universal search in the framework files for each of those hooks and you can see what actions (or even additional hooks) are attached to them.
All in all, there are over 50 hooks in the Genesis Framework that enable you to inject custom code almost anywhere on the page. StudioPress has put together detailed documentation on each of these hooks, along with examples of use, here (available to members only).
Making customisations
Just like with the WordPress core, you should never directly edit files from the Genesis Framework. Always make your customisations via a child theme (we'll talk more about this in a bit). If you make edits to the actual framework, they'll be lost the next time you apply an update. If you stick to working with a child theme, your customisations are safe from being overwritten and you can update Genesis anytime an update is available.
If you're 'tinkering' with code customisations, you'll do the vast majority of your work in the child theme's 'functions.php' file. From there you can universally apply code (e.g. remove post meta throughout the whole site), or combine your functions with WordPress conditional statements to target specific content (e.g. remove post meta on any posts in the 'Comedy' category). You can also overwrite any default Genesis functionality by creating specific template files. For example, if you have a custom post type for 'Movies' , you can use the standard WordPress template hierarchy to create an archive or single template file to display your movies.
My rule of thumb for whether to use 'functions.php' or a custom template file is how many customisations I'll make. For instance, if I can write a handful of functions to do what I need, I'll just add those to 'functions.php'. If it's more complex than that, I'll consider using a custom template.
For style changes, I recommend going directly to 'style.css'. Since you never need to update child themes (that's the point of using them), you can directly edit the style sheet or any other file and make it yours. Really, there's no right or wrong way to add your customisations. It's more about learning the most efficient way to get the results you want, and following good coding principles as you go.
Child themes
So far we've talked about the basics of Genesis and customisations. Now let's look at child themes. First off, Genesis child themes cannot be used on their own. The Genesis Framework must also be installed (but not activated) on your WordPress site. From there, you can activate any Genesis child theme. As with the traditional WordPress parent/child theme structure, a Genesis child theme automatically inherits everything from the parent framework. You can think of a child theme as a way to remove the things you don't want from Genesis and add in any extra bells and whistles that you do. Also, the child theme is where all of your custom styles are. Genesis itself includes very little CSS, as it's not intended for use as a standalone theme.
If you want as basic a theme as possible, start with the Genesis Sample theme, available for free on GitHub (or in your StudioPress account, if you have one). The theme is visually very sparse, so it's a good place to begin making both style changes and code changes. I've found the best way to learn is to open up the 'functions.php' of your child theme and start experimenting with code. My favourite starting point is to look at 'post.php' in the Genesis source code and pick any add action from the genesis_reset_loops() – the function that spits out the guts of a post, from the image at the top to the comments section at the bottom.
Since we already talked about post meta, I'll copy that add_action() statement and paste it into 'functions.php':
add_action( 'genesis_entry_footer', 'genesis_post_meta' );
Next, change the add to remove. This reverses, or overrides what Genesis would output on its own.
remove_action( 'genesis_entry_footer', 'genesis_post_meta' );
That's a simple example and probably not practical (it removes the post meta everywhere on your site, including your blog page, archive pages and single posts), but it demonstrates how you can work with Genesis via your child theme.
Don't be timid about trying things out in your development environment. You may white-screen it a bit, but experimentation really is the best way to get comfortable with the framework (or any code, for that matter). I also recommend using Google liberally. There are hundreds, if not thousands, of Genesis tutorials out there. There are even formal courses available at both Lynda.com and Treehouse.
In conclusion
If you're new to theme development, you'll find Genesis is very approachable, especially considering the bounty of free learning resources online. If you've already spent some time in WordPress theme development, you'll have a definite learning curve with Genesis, but once you're over the hump, you'll love what you can do with it.
Used by over 130,000 folks and under active development, Genesis isn't going anywhere any time soon. If you plan to stick around in the WordPress theme industry, it's definitely worth a look. Who knows, it might become your favourite tool for building websites.
Words: Carrie Dils
Carrie Dils is WordPress developer and consultant. This article originally appeared in issue 268 of net magazine.
Liked this? Read these!
- 9 top WordPress resources
- Brilliant Wordpress tutorial selection
- Free tattoo fonts for designers
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
Daily design news, reviews, how-tos and more, as picked by the editors.
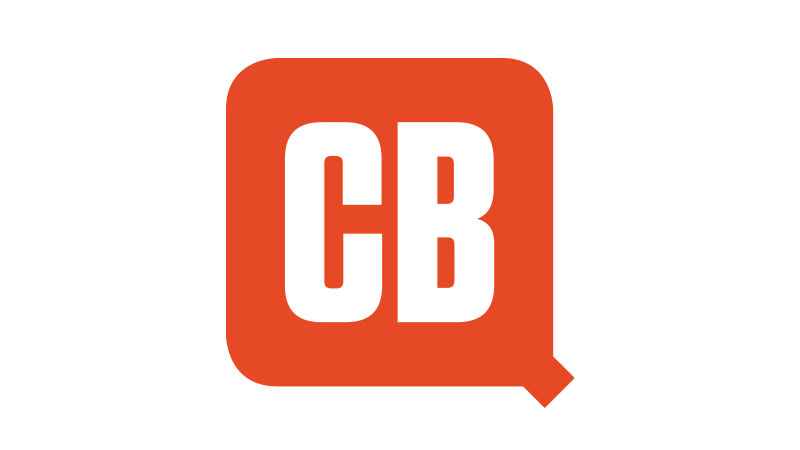
The Creative Bloq team is made up of a group of art and design enthusiasts, and has changed and evolved since Creative Bloq began back in 2012. The current website team consists of eight full-time members of staff: Editor Georgia Coggan, Deputy Editor Rosie Hilder, Ecommerce Editor Beren Neale, Senior News Editor Daniel Piper, Editor, Digital Art and 3D Ian Dean, Tech Reviews Editor Erlingur Einarsson, Ecommerce Writer Beth Nicholls and Staff Writer Natalie Fear, as well as a roster of freelancers from around the world. The ImagineFX magazine team also pitch in, ensuring that content from leading digital art publication ImagineFX is represented on Creative Bloq.