How to use Velocity to easily add animations
Velocity.js is a free, lightweight library that you should be using - developer Neal O'Grady gets you started.
Motion attracts our attention; it's an innate survival mechanism that served our ancestors well for thousands of years. It allowed them to detect predators sneaking up on them. Nowadays, it's mostly a leftover instinct that us web designers use to command the attention and interest of users on our sites.
Adding animation and transitions such as opacity fades, color changes, scaling, and 3D motion, turns an otherwise flat, static collage of words and images into a dynamic and interesting site. You should absolutely be adding animations to your website designs – when applied sparingly and with purpose, it makes them better.
But how can you do this exactly? There are two ways to add animation to a site, with CSS and with JavaScript.
CSS is perfect for small sites that don't require much custom coding, and for simple user interactions, like small stylistic changes for elements' hover states. (Or if you simply have no desire to do any programming!)
For anything beyond these simple interactions, the best way to add animation to a site is with JavaScript, and the best modern animation library for doing this is Velocity.js.
This article is unique in that it's going to take a ground-up approach to learning web animation. If you're a web designer who hasn't dabbled much into JavaScript but always wanted the power of advanced animation, then keep on reading.
What is Velocity.js?
Velocity is a free, lightweight library that lets you easily add animations to your sites, ranging from the simplest of animations to the most complex. Velocity outperforms all other animation libraries, is simple to use, and mimics the syntax of the most popular JavaScript library, jQuery. It's also well supported by all browsers and devices, and has been adopted by major companies like Uber and WhatsApp.
Daily design news, reviews, how-tos and more, as picked by the editors.
Velocity is best used with jQuery, but it doesn't have to be. What is jQuery? jQuery is a JavaScript library designed to simplify selecting and manipulating HTML elements. It has practically become assumed that jQuery is used on most web project – it's that popular.
jQuery has its own animation tools exposed via its "animate" function, but because of how monolithic jQuery is, it produces choppy animations. It's also not nearly as feature rich as Velocity. Velocity allows you to leverage the element selection power of jQuery, and skip past jQuery's animation shortcomings. The difference, especially to establishing an easy animation workflow, is night and day.
Velocity offers some great advantages over both CSS and jQuery based animations, which I'll cover after we've gone through the basics of using it. To give you a preview of what's to come, these benefits include scrolling animations, animation reversals, physics-based motion, and animation chaining. Some pretty cool stuff.
For now, let's get started on how to use it.
How do I use Velocity?
The first step is to download the code from the Velocity website (copy-paste the code into a text-editor, and save it as "velocity.min.js"). Alternatively, you can pull it directly into your HTML (as shown in the code example below).
Either way, include the Velocity file using a <script> tag before the closing body tag of your document, and before the JavaScript file you'll be coding in ("script.js"):
<html>
<head>
…
</head>
<body>
…
<script src="//code.jquery.com/jquery-2.1.1.min.js"></script>
<script src="//cdn.jsdelivr.net/velocity/1.1.0/velocity.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Note: If you are using jQuery, make sure that you include jQuery before Velocity. Velocity adapts itself given the presence of jQuery.
Once the code is included in a script tag, you can start using the velocity() function throughout your "script.js" file.
How do I run this function?
You use the Velocity function on a jQuery element (assuming you're using jQuery). For example, say you have the following paragraph you wish to animate:
<p id="example">
This is an example element you can select and animate.
</p>
We can select it with jQuery with the paragraph's ID ("example"), and store it into a variable:
var $element = $("#example");
This creates a jQuery element object named $element that represents this paragraph. We can now run velocity function calls on this element by using this $element variable:
$element.velocity( … some command here … );
Okay, so what are the arguments it accepts?
Arguments
Velocity accepts one or more arguments. The first argument is mandatory. It can either be the name of one of the predefined commands (examples to follow), or an object of different CSS properties that should be animated.
// Object defining the animation's final values of width (50%), and left (500px)
$element.velocity({ width: "500px", left: "500px" });
Note: The order of properties in a data object are irrelevant. Everything in the animation happens at the exact same time.
The second argument, which is optional, is also an object. It contains animation options such as duration, easing, and delay, and complete (a function that executes after the animation completes):
// Animates an element to a width of 300px over 500ms after a 1000ms delay.
$element.velocity({ width: "300px" }, { duration 500, delay: 1000 });
Unlike in CSS, where you can pass in multiple numeric values into a CSS property like margin (margin: "10px 5px 8px 12px"), with Velocity you must use a single value per CSS property. Therefore, you must set each component separately: { marginRight: "10px", marginTop: "5px" … }.
This may seem like a handicap, but it's actually a benefit. Not only is this more legible and explicit, it also allows you to set individual easing types for each sub-property, rather than being forced to have one easing type for all of them. This gives you direct control to more pieces of your animation!
Note: CSS properties containing multiple words (margin-left and background-color) cannot be hyphenated, but must be written using camelcase (marginLeft and backgroundColor).
Property values
If you don't provide a unit type, Velocity will assume one for you (normally ms, px, and deg). Nevertheless, I would recommend being explicit so you can discern the unit types at a glance whenever you or a co-worker looks back through your code. If the value contains anything other than a numerical value (%, or letters), then you have to use quotes.
// Okay, only numerical value
$element.velocity("scroll", { duration: 500 })
// Okay, uses quotes
$element.velocity("scroll", { duration: "500ms" })
// Not okay, contains "ms" without quotes
$element.velocity("scroll", { duration: 500ms })
What is this "easing" business?
I've used the word easing a few times so far, and perhaps you're confused as it what that means. Easings determine the speed of an animation at different stages throughout its duration. For example, an "ease-in" easing gradually accelerates at the beginning of the animation and then remains constant until it ends. An "ease-out" easing starts at a linear speed, and gradually decelerates near the end of the animation. A "linear" easing has a constant speed throughout the duration, and looks very jarring and robotic.
Conveniently, you specify easing with the "easing" option:
// Animate an element to a width of 500px with an easing of "ease-in-out"
$element.velocity({ width: 500 }, { easing: "ease-in-out" });
Easings get much more complicated, but for brevity's sake, I'll stop here. Read Velocity's documentation for more information.
Chaining
Creating a series of sequential animations in CSS requires manually calculating timing delays and durations for each individual animation. And if any one of these steps needs to be changed, all the animations proceeding this step must be recalculated and changed as well.
Velocity allows for simple chaining of animations one after another, just by calling the velocity function one after the other:
$element
// Animates the element to a height of 300px over 1000ms
.velocity({ height: 300 }, { duration: 1000 })
// Animates the element to a left position of 200px over 600ms after the width is finished animating
.velocity({ top: 200 }, { duration: 600 })
// Fades the element over 200ms out after it is done moving
.velocity({ opacity: 0 }, { duration: 200 });
Here's a Codepen example of chaining.
Note: You will notice that only the last velocity call has a ";" at the end of the line. This is extremely important. To chain together animations, all the "velocity" calls must be performed together on the same element, and you cannot terminate the command line using a semicolon. JavaScript ignores whitespace, so these calls would look like this: $element.velocity(...).velocity(...).velocity(...).
Back to the features
That should give you a peek into Velocity and how to use it. Now that you won't be overwhelmed, let's go back to describing those cool benefits it provides over CSS and jQuery.
Page scrolling
Single-page sites are trending in web design now, where a site is divided into sections, rather than into separate pages. As a result, clicking on a navigational link simply causes the page to scroll down to the appropriate page section. Without an animation, it's an instantaneous and jarring jump, providing the user with no context as to where this content is located relative to the rest of the page.
CSS cannot perform scrolling, so this is one of the most popular uses for JS animations.
To perform scrolling with Velocity, simply run the "velocity" function with the "scroll" command on the element you wish to scroll to:
$element.velocity("scroll", { duration: 1200 });
This calls causes the browser to scroll down to the top edge of the selected element over 1200ms. With jQuery alone, this would be a much more complicated, multi-line function. I'll spare you the confusion induced headache by not including it here.
Here's a Codepen example of scrolling.
Animation reversal
In jQuery, to revert back to the element's original state before the animation started, you have to manually animate its properties back to their original values. For example:
With jQuery:
// Animate a 100px tall element to a height of 200px over 500ms
$element.animate({ height: "200px" }, { duration: 500 });
// Animate the element back to its original height value of 100px over 500ms
$element.animate({ height: "100px" }, { duration: 500 });
With Velocity, however, you just have to run the reverse command:
// Animate a 100px tall element to a height of 200px over 500ms
$element.velocity({ height: "200px" }, { duration: 500 });
// Reverse previous animation – animate back to the original height of 100px over 500ms
$element.velocity("reverse");
This previous calls reverse the animation to the selected element's original state prior to the animation—no need to specify the values manually.
Here's a Codepen example of animation reversal.
Physics-Based Motion
The real world isn't perfect, or smooth – neither is real-world motion. It has fast parts, slow parts, weight, momentum, and friction. Unlike with CSS, you can mimic real-world physics in your JS-based animations, making things appear more natural. Linear motion looks robotic (lifeless), and jarring.
To allow for realistic motion, Velocity accepts an easing based on spring physics. This easing type takes an array of a tension value (default: 500) and a friction value (default: 20) as its parameter (see Velocity documentation for more information).
// Animate the selected element to a height of 200px over the default 400ms using a spring physics easing. Spring tension is set to 250, and friction is set to 10.
$element.velocity({ height: "200px", { easing: [ 250, 10 ] });
A high tension value increases total speed and bounciness of the animation. A high friction value causes the animation to decelerate faster, reducing it's speed near the end of the animation. Tweaking these values will make each animation unique, realistic, and interesting.
The example animation above would be slow and stiff (low tension), and would only slowly decelerate throughout the duration (low friction).
Here's a Codepen example using spring physics.
Grande finale
Okay, maybe you need a couple examples of real animations and Velocity commands. Let's start with animating a box's width and height, combining both chaining and animation reversal, and let's use the following box element:
<div id="box">
</div>
With the following CSS styling:
#box {
width: 100px; // Set box width to 100px
height: 100px; // Set box height to 100px
border: solid 1px #000; // Give the box a 1px solid black border to make it visible
}
You could animate its width, followed by its height, and then revert it back to its original dimensions with the following velocity calls:
// Select the box element by ID
$("#box")
// Animate to a width of 200px over 500ms
.velocity({ width: 200px }, { duration: 500 })
// Animate to a height of 50px over 300ms directly after width stops animating
.velocity({ height: 50px }, { duration: 300 })
// Animate back to original width and height after height stops animating
.velocity("reverse");
And now let's add one of the most useful, yet easy to add, features: creating navigational links that scroll down to the appropriate page section.
Say you have the following navigational bar and page sections:
<nav>
<a class="nav-link" href="#product">Product</a>
...
<a class="nav-link" href="#about">About</a>
</nav>
<div id="#product">
…
</div>
...
<div id="#about">
...
</div>
We want to add the scroll animation to every navigational link's (class of "nav-link") click event, which will cause the page to scroll down to the appropriate page section whose ID is contained in the link's href.
// Attach a click event to the "nav-link" class
$(".nav-link").on("click", function() {
// Grab the target page section's ID from the link's (contained within "this") href attribute
var scrollTargetID = $(this).attr("href");
// Select this element by ID using jQuery
var $scrollTarget = $(scrollTargetID);
// Add the scroll animation to the element
$scrollTarget.velocity("scroll", { duration: 1000 });
});
There's a couple examples of how to use velocity on actual page elements, for more examples, check out Velocity's documentation.
Now go out and make the web dynamic
Animations attract our attention and breathe life into an otherwise static page, and JavaScript is the best way to add them to your projects – and Velocity is the best JavaScript animation library out there. That's why I wrote this article.
The improvement and expansion of CSS animations is limited by the infrequent updates of the CSS standard. With JavaScript, the open-source community produces dozens of new libraries, plugins, and updates every single day – widening the horizons of your animations through the invention of new tools.
Velocity has many more features than those demonstrated here, and I encourage you to check out its documentation. Now go experiment!
Words: Neal O'Grady
Neal O'Grady is an Irish-Canadian freelance web developer, designer, and writer. He's written on design topics for the Webflow blog, and on random thoughts on his personal blog.
Like this? Read these!
- Brilliant Wordpress tutorial selection
- How to start a blog
- The designer's guide to working from home
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
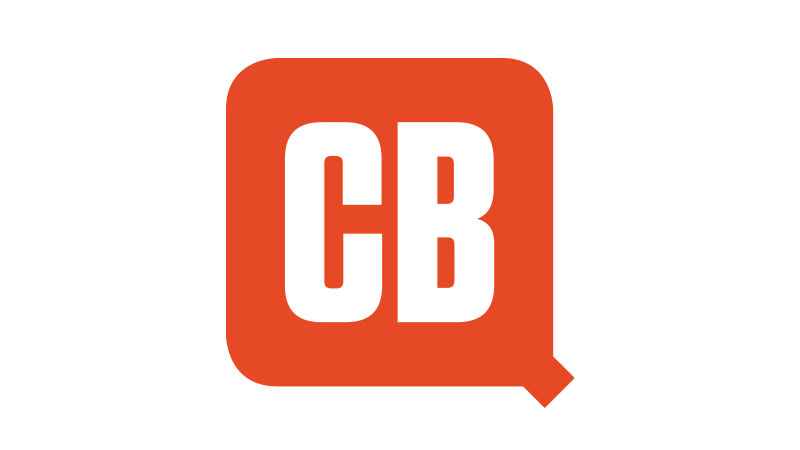
The Creative Bloq team is made up of a group of art and design enthusiasts, and has changed and evolved since Creative Bloq began back in 2012. The current website team consists of eight full-time members of staff: Editor Georgia Coggan, Deputy Editor Rosie Hilder, Ecommerce Editor Beren Neale, Senior News Editor Daniel Piper, Editor, Digital Art and 3D Ian Dean, Tech Reviews Editor Erlingur Einarsson, Ecommerce Writer Beth Nicholls and Staff Writer Natalie Fear, as well as a roster of freelancers from around the world. The ImagineFX magazine team also pitch in, ensuring that content from leading digital art publication ImagineFX is represented on Creative Bloq.